07. Buffered Streams
Buffered Streams
In this section, you will learn how to improve your code's efficiency by using buffered streams.
ND079 JPND C2 L02 A10 Buffered Streams V2
What Are Buffered Streams?
The most common buffered streams are BufferedReader
s and BufferedWriter
s, which read and write lines of text.
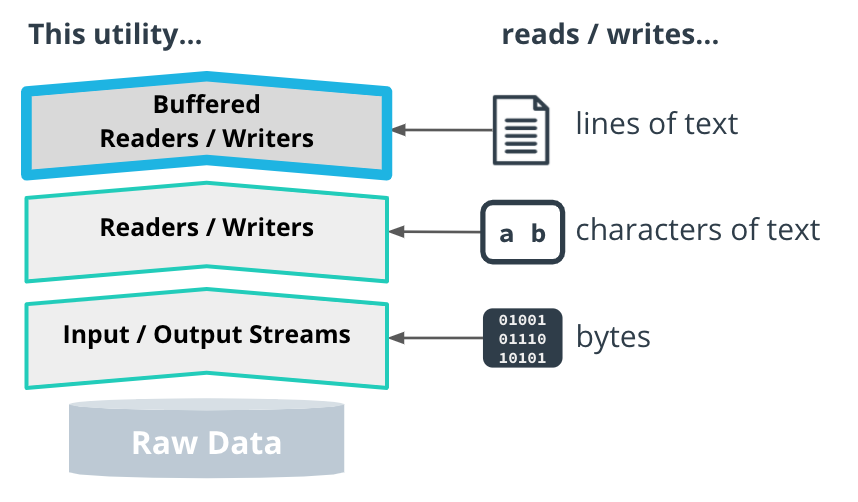
BufferedReaders and BufferedWriters Work with Lines of Text
QUIZ QUESTION::
Match each Input/Output utility with its description
ANSWER CHOICES:
Input/Output |
Description |
---|---|
Input/Output Streams |
|
Readers/Writers |
|
Buffered Readers/Writers |
SOLUTION:
Input/Output |
Description |
---|---|
Readers/Writers |
|
Input/Output Streams |
|
Buffered Readers/Writers |
Why Do We Use Buffered Streams?
Buffered streams reduce the number of I/O operations performed by your program. This can significantly shrink the amount of time your program spends doing I/O!
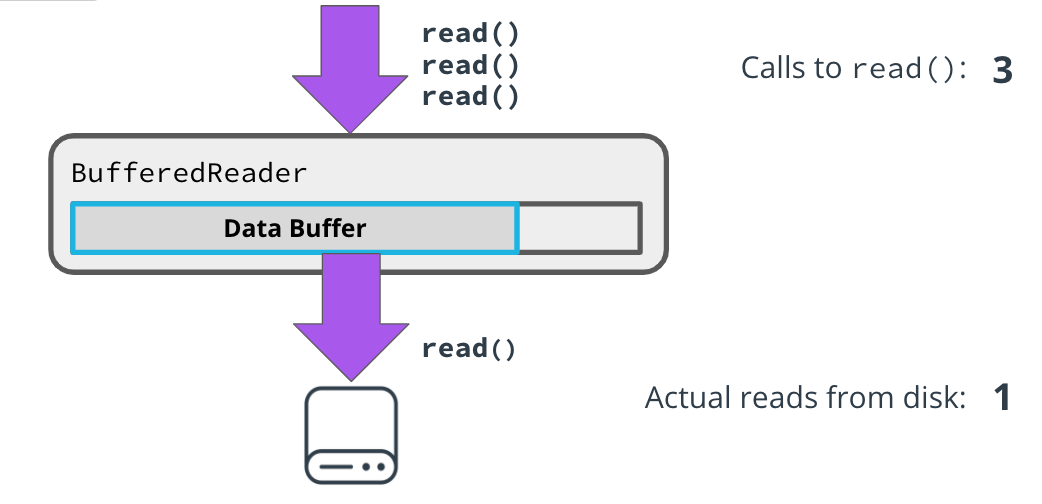
Using A BufferedReader Can Reduce Your Reads from Disk
When your code calls BufferedReader.read()
, the BufferedReader
reads ahead, and fetches more data than you asked for. Whatever it reads is stored in an array, which is also called a buffer.
The next time you call read()
, if the data you requested is already in the buffer, the BufferedReader will give you that cached data, without having to do another read from disk!
SOLUTION:
600 bytesBufferedReader
Example
BufferedReader reader =
Files.newBufferedReader(Path.of("test"), StandardCharsets.UTF_8);
String line;
while ((line = reader.readLine()) != null) {
useString(line);
}
reader.close();
From an API perspective, you already know how to create BufferedReader
s. In fact, the Files API only returns buffered readers.
The main difference between this API and the Reader
API is the addition of the readLine()
method, which returns a full line of text. Reading lines this way is a lot easier than reading the individual characters one by one!
SOLUTION:
Creates an array of pointers to disk.BufferedWriter
Example
BufferedWriter writer =
Files.newBufferedWriter(Path.of("test"),
StandardCharsets.UTF_8);
writer.write("Hello, ");
writer.write("world!");
writer.flush(); // Writes the contents of the buffer
writer.close(); // Flushes the buffer and closes "test"
BufferedWriter
also uses an in-memory buffer to store writes, and then periodically writes contents of the buffer in batches.
In this code, the write()
method is called twice, but there is only one actual write to the underlying output stream.
Flushing
Emptying out the buffer is called flushing. You can force this to happen immediately by calling the flush()
method. If you are writing a file, flushing the buffer will write its contents to the file so that any changed become visible.
SOLUTION:
- `readLine()`
- `flush()`